COBOL in the 21st Century
Language
- unknown
by James Smith (Golang Project Structure Admin)
This blog post will attempt to provide a basic introduction to the COBOL programming language for complete beginners.
We will cover the language’s history, syntax, features and applications. Then we will provide some practical examples to help you understand better how COBOL code works.
Table of Contents
What Is COBOL?
Common Business-Oriented Language, known usually as COBOL, is a programming language that was first developed in the late 1950s.
Designed during an era when computers were just beginning to revolutionize business processes, COBOL quickly became the backbone of many critical operations.
COBOL is known for its readability, simplicity and longevity — traits that have made it accessible to programmers and business professionals alike.
Despite its impressive age, COBOL remains remarkably relevant. Some of the world’s largest organizations still rely on it to power their core systems, a testament to its well-proven robustness and the enduring trust it has earned over more than half a century
What are the Most Common Applications of COBOL?
COBOL has primarily been used for business applications such as accounting, payroll and inventory management. It is also used in the finance sector for applications such as banking and insurance.
The COBOL programming language is particularly well-suited for applications that require high levels of accuracy and reliability, since it is well known for its robustness and stability.
COBOL is very often used in legacy systems that were developed decades ago. Many large organizations still use COBOL to maintain these systems, because it is often more cost-effective to continue using existing systems rather than replacing them with newer technology.
What Is the History of COBOL?
COBOL began being developed towards the end of 1950s by a committee of computer scientists, businesspeople and government officials under the auspices of the US Department of Defense.
The goal of the committee was to create a programming language that could be used by non-technical business professionals to write their own programs. At the time, most programming languages were designed for scientific or mathematical applications, which meant that they were not well-suited to the needs of the business world.
The first version of COBOL was released in 1960, and it very quickly became popular among businesses and government agencies. By the 1970s, COBOL had become the dominant programming language for business applications, and it remained so for several decades.
Did Grace Hopper Contribute to COBOL?
Grace Hopper was a pioneering American computer scientist and U.S. Navy officer who made groundbreaking contributions to the field of computing.
She is best known for her work in developing one of the first ever compilers, a tool that translates human-readable code into machine code, which paved the way for modern programming languages.
Hopper played a key role in the creation of COBOL, which is closely tied to her earlier work on FLOW-MATIC, the first programming language to use English-like commands.
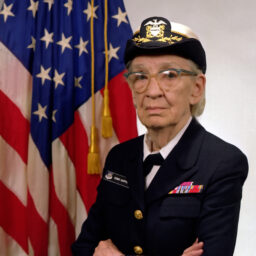
Developed in the early 1950s for the UNIVAC I computer, FLOW-MATIC was groundbreaking precisely because it allowed programmers to write instructions in a language that resembled plain English, making it easier for non-specialists to understand.
Hopper believed that programming languages should be machine-independent and accessible to business users, a philosophy she would later carry into the development of COBOL.
How Does the Syntax of COBOL Look?
COBOL syntax is based on English-like phrases and sentences, which makes it relatively easy to read and understand compared to more abstract programming languages.
In COBOL, each program is divided into sections, paragraphs and sentences, which are arranged in a specific order.
Below is an example of a very simple COBOL program:
Hello, World!
IDENTIFICATION DIVISION.
PROGRAM-ID. FIRST-EXAMPLE.
PROCEDURE DIVISION.
DISPLAY 'HELLO, WORLD!'.
STOP RUN.
In this program, the first section is the IDENTIFICATION DIVISION
, which contains information about the program, such as its name and author. The second section is the PROCEDURE DIVISION
, which contains the instructions that the program will execute.
The DISPLAY
statement is used to print a message to the screen, and the STOP RUN
statement is used to end the program. Note that each statement ends with a period, and the program as a whole ends with the STOP RUN
statement.
The indentation that can be seen before the PROGRAM-ID
, DISPLAY
and STOP RUN
statements is not explicitly required by COBOL, but it’s a common practice to improve readability. Indentation helps to visually separate different sections within the code.
What Are Some of the Best Features of COBOL?
COBOL has several features that make it particularly well-suited to business applications.
One of the most important features is its support for decimal arithmetic, which is essential for financial calculations.
In addition, COBOL has built-in support for file handling, which makes it easy to read and write data to and from disk.
The language also has a rich set of data types, including alphanumeric, numeric and date types. And it has a powerful string manipulation capability, which makes it easy to work with text data.
COBOL also supports arrays, which are useful for storing large amounts of data.
Looking at Some Example Programs Written in COBOL
Below are four examples of simple COBOL programs that demonstrate the programming language’s basic syntax and features.
Simple Arithmetic Calculation
IDENTIFICATION DIVISION.
PROGRAM-ID. ARITHMETIC.
DATA DIVISION.
WORKING-STORAGE SECTION.
01 A PIC 9(2) VALUE 10.
01 B PIC 9(2) VALUE 5.
01 C PIC 9(2).
PROCEDURE DIVISION.
COMPUTE C = A + B.
DISPLAY 'A + B = ', C.
COMPUTE C = A - B.
DISPLAY 'A - B = ', C.
COMPUTE C = A * B.
DISPLAY 'A * B = ', C.
COMPUTE C = A / B.
DISPLAY 'A / B = ', C.
STOP RUN.
This program begins by identifying itself with the title ARITHMETIC.
Then we see the DATA DIVISION
, which is used to define all of the variables, constants and storage areas required by the program. Here the WORKING-STORAGE SECTION
defines three variables:
A
: a two-digit numeric variable,PIC 9(2)
, which is initialized to the value10
. ThePIC 9
part of the statement refers to the fact that the variable will hold numeric values between 0 and 9. The wordPIC
is an appreciation for picture, and it just means that the variable will hold a string of characters, and in this case these characters will all be digits.B
: a two-digit numeric variable,PIC 9(2)
, which is initialized to the value5
.C
: a two-digit numeric variable,PIC 9(2)
, that will be used to hold the result of the subsequent arithmetic operation. This is given no initialization value.
Finally, when the various calculations (adding, subtracting, multiplying and dividing A
and B
) are performed with the COMPUTE
statement, the DISPLAY statement is used after each calculation to print the result, which is stored in the C
variable, to the screen.
The STOP RUN
statement can ordinarily be seen at the end of all COBOL programs. It terminates the program’s execution and returns control back to the operating system.
Reading and Writing to a File
IDENTIFICATION DIVISION.
PROGRAM-ID. FILE-IO.
DATA DIVISION.
FILE SECTION.
FD INPUT-FILE.
01 INPUT-RECORD.
05 EMPLOYEE-NAME PIC X(20).
05 SALARY PIC 9(5).
WORKING-STORAGE SECTION.
01 WS-COUNT PIC 99 VALUE 0.
PROCEDURE DIVISION.
OPEN INPUT INPUT-FILE.
READ INPUT-FILE INTO INPUT-RECORD.
PERFORM UNTIL (INPUT-FILE AT END)
ADD 1 TO WS-COUNT.
DISPLAY 'EMPLOYEE NAME: ', EMPLOYEE-NAME.
DISPLAY 'SALARY: ', SALARY.
READ INPUT-FILE INTO INPUT-RECORD.
END-PERFORM.
CLOSE INPUT-FILE.
DISPLAY 'TOTAL NUMBER OF RECORDS: ', WS-COUNT.
STOP RUN.
This COBOL program, named FILE-IO
, demonstrates how to read data from a file and display its contents on the screen. It also counts the number of records processed and displays the total number at the end of execution.
The FILE SECTION
, within the DATA DIVISION
, defines the structure of the file being read.
The FD INPUT-FILE
statement specifies that the program will handle a file called INPUT-FILE
. The FD
keyword stands for file description, and it used to declare the file’s logical name.
The INPUT-RECORD
structure defines how the records in the file will be read. In this case, each record contains two fields.
The first field is EMPLOYEE-NAME
and it consists of alphanumeric characters, PIC X(20)
, that can hold up to 20 characters for the employee’s name.
The second field is SALARY
and it is a numeric string, PIC 9(5)
, that holds up to 5 digits representing the employee’s salary.
The WORKING-STORAGE SECTION
, as we saw in the previous example, is where intermediate variables used in the program are declared.
In our example, only one intermediate variable, named WS-COUNT
, is declared. This is a two-digit numeric variable, PIC 99
, initialized to 0
. It is used to count the number of records processed during the execution of the program.
The PROCEDURE DIVISION
contains the actual program logic — the sequence of instructions that will be executed when the code runs.
The OPEN-INPUT
statement opens the INPUT-FILE
for reading. In COBOL, before any file operations can be performed, the file must be explicitly opened with the OPEN
statement. Here the file is opened in INPUT
mode, meaning it is opened for reading only.
Next the READ
statement reads the first record from the INPUT-FILE
and stores the record in the INPUT-RECORD
structure. The data is broken down into the two fields, EMPLOYEE-NAME
and SALARY
, based on the file’s structure, which was defined earlier.
The PERFORM-UNTIL
loop continues iterating until the INPUT-FILE
reaches the end of the file, which is determined by an internal condition (AT END
) that becomes true when there are no more records to read.
Each time a record is read, the variable WS-COUNT
is incremented by 1, as can be seen on the first line within the loop. This keeps track of how many records have been processed.
Next two DISPLAY
statements print the employee’s name and salary to the screen.
The statement at the end of the loop reads the next record from the file, ready for the next iteration, repeating the process until the end of the file is reached.
Once all records have been processed, the file is closed with the CLOSE
statement. Closing the file makes clear that the program has finished using the file and releases any resources associated with it.
After all of the records have been processed, the total number of records read, stored in WS-COUNT
, is displayed on the screen. This gives the user an understanding of how many records were processed.
String Manipulation
IDENTIFICATION DIVISION.
PROGRAM-ID. STRING-EXAMPLE.
DATA DIVISION.
WORKING-STORAGE SECTION.
01 NAME-IN PIC X(20) VALUE 'JOHN DOE'.
01 NAME-OUT PIC X(100).
PROCEDURE DIVISION.
STRING 'HELLO, MY NAME IS '
NAME-IN ' AND I AM '
'30 YEARS OLD.' DELIMITED BY SIZE
INTO NAME-OUT.
DISPLAY NAME-OUT.
STOP RUN.
This COBOL program, named STRING-EXAMPLE
, showcases how to concatenate multiple strings, both fixed and variable, using the STRING
statement. The final result is stored in a variable and then displayed to the user using the DISPLAY
statement.
In the WORKING-STORAGE SECTION
, two variables are declared.
The first, NAME-IN
, is an alphanumeric variable, PIC X(20)
, that can hold up to 20 characters. This variable is initialized with the value 'JOHN DOE'
. Because this string is shorter than 20 characters, the compiler will fill the unused characters with spaces.
The second, NAME-OUT
, is another alphanumeric variable, PIC X(100)
, which will be used to store the final concatenated result. It is declared but not initialized here, meaning that it will be populated during the program execution.
The STRING
statement in COBOL is used to concatenate multiple strings or variables into one destination variable.
Within this statement, the first part of the string, 'HELLO, MY NAME IS '
, is a fixed string. This text will be the starting point of the final output. The second part of the string is the NAME-IN
variable, which contains the value 'JOHN DOE'
. The program inserts the value of this variable into the output.
After NAME-IN
, the program continues with more fixed strings: ' AND I AM '
and '30 YEARS OLD'
.
The words DELIMITED BY SIZE
tell COBOL to concatenate the strings based on their entire size, ensuring that all characters in each string and variable are included in the final output. No explicit delimiter like a space or comma is used here; the strings are concatenated in the order provided.
The concatenated string that results is placed into the NAME-OUT
variable. This variable will now contain the final string. It is printed out to the screen with the DISPLAY
statement.
Calculating Employee Salaries
IDENTIFICATION DIVISION.
PROGRAM-ID. EMPLOYEE-SALARIES.
DATA DIVISION.
WORKING-STORAGE SECTION.
01 RECORDS OCCURS 10 TIMES INDEXED BY I.
05 EMPLOYEE-NAME PIC X(20).
05 HOURS-WORKED PIC 9(3) VALUE 0.
05 HOURLY-RATE PIC 9(5)V99 VALUE 0.00.
05 WEEKLY-SALARY PIC 9(6)V99 VALUE 0.00.
01 TOTAL-HOURS PIC 9(5) VALUE 0.
01 TOTAL-SALARY PIC 9(7)V99 VALUE 0.00.
01 AVERAGE-SALARY PIC 9(7)V99 VALUE 0.00.
PROCEDURE DIVISION. MAIN-PROCEDURE.
PERFORM VARYING I FROM 1 BY 1 UNTIL I > 10
DISPLAY 'ENTER EMPLOYEE NAME: '
ACCEPT EMPLOYEE-NAME(I)
DISPLAY 'ENTER HOURS WORKED: '
ACCEPT HOURS-WORKED(I)
DISPLAY 'ENTER HOURLY RATE: '
ACCEPT HOURLY-RATE(I)
COMPUTE WEEKLY-SALARY(I) = HOURS-WORKED(I) * HOURLY-RATE(I)
ADD HOURS-WORKED(I) TO TOTAL-HOURS
ADD WEEKLY-SALARY(I) TO TOTAL-SALARY
END-PERFORM.
COMPUTE AVERAGE-SALARY = TOTAL-SALARY / 10
DISPLAY 'TOTAL HOURS WORKED: ', TOTAL-HOURS
DISPLAY 'TOTAL SALARY PAID: ', TOTAL-SALARY
DISPLAY 'AVERAGE SALARY: ', AVERAGE-SALARY
STOP RUN.
This COBOL program, named EMPLOYEE-SALARIES
, is designed to calculate the weekly salaries of 10 employees based on their hours worked and hourly rate. In addition to determining each employee’s weekly salary, the program computes the total hours worked, the total salary paid to all employees and their average salary.
At the core of the program is the WORKING-STORAGE SECTION
, which defines the variables and data structures necessary for performing these calculations. The RECORDS
data structure uses the OCCURS
clause to define an array that can hold information for 10 employees. Each record includes each employee’s name, hours worked, hourly rate and weekly salary. The array is indexed by the variable I
, which allows the program to loop through each employee’s data one by one.
Note that the PIC 9(5)V99
format is used for the HOURLY-RATE
, WEEKLY-SALARY
, TOTAL-SALARY
and AVERAGE-SALARY
variables. The V
in the PIC
clause represents an implicit decimal point, allowing COBOL to handle decimal values for currency without requiring explicit decimal points in the data entry. This feature is essential for handling monetary values accurately, ensuring that calculations like salary can be done with two decimal places.
As in previous examples, the program’s main logic resides in the PROCEDURE DIVISION
, where it uses the PERFORM
statement to iterate through the 10 employees.
For each employee, the program prompts the user to enter the employee’s name, the number of hours worked, and their hourly rate. The input is stored in the respective elements of the RECORDS
array.
The program then computes each employee’s weekly salary by multiplying the hours worked by the hourly rate using the COMPUTE
statement. This computed value is stored in the WEEKLY-SALARY
field for that particular employee.
In addition to calculating the salary for each employee, the program simultaneously accumulates the total number of hours worked and the total salary paid by using the ADD
statement. These totals are updated for each employee processed, allowing the program to maintain running sums of both metrics throughout the loop.
Once all 10 employees have been processed, the program computes the average salary by dividing the total salary by 10. The results of the calculations are then displayed using the DISPLAY
statement, which prints the total hours worked, total salary paid and the average salary.
How Is COBOL Used in the 21st Century?
COBOL is still being used in the 21st century, primarily by large organizations that have legacy systems developed decades ago. These organizations have invested heavily in their existing systems and so find it more cost-effective to maintain them than to replace them with new technology.
In addition, efforts are underway to modernize existing COBOL systems by integrating them with newer technology. For example, some organizations are using COBOL programs as web services, allowing them to be accessed by modern web applications. Other organizations are using software tools to automatically translate COBOL code into newer programming languages, making it easier to maintain and update existing systems.
How is the Job Market for COBOL Developers?
While the demand for professional programmers with knowledge of COBOL had been declining, the COVID-19 pandemic has highlighted the importance of maintaining these systems.
The job market for COBOL programmers has been experiencing somewhat of a resurgence in recent years due to the critical need, as discussed above, for maintaining and modernizing legacy systems.
According to job market data, there has been an increase in job postings for COBOL programmers in recent years. Many of these job openings are for positions that require experience in maintaining and modernizing legacy systems.
In addition, there has been a shortage of experienced COBOL programmers, as many of the existing workforce are nearing retirement age. This, of course, has created an opportunity for younger developers to enter the job market and develop their skills in COBOL.
What Can a COBOL Programmer Expect to Earn?
The average salary for COBOL programmers varies widely depending on location and the specific job role.
In general, COBOL programmers can expect to earn a competitive salary, particularly if they have extensive experience in maintaining and modernizing legacy systems.
Moreover, in some cases, COBOL programmers with a strong track record have been able to earn more than $100 per hour (according to an article published in 2017).