Companies Using Go Code in Production Right Now
Language
- unknown
by James (Golang Project Structure Admin)
We spend a lot of time on this blog thinking about things like syntax and code style, or building example projects, but today I want to zoom out and look at Go programming from a bigger perspective.
Who Uses Go in Real-World Applications?
Since Go is one of the most popular languages being used in GitHub repositories, I thought it would be interesting to explore in this post how some major companies and global corporations have used the Go programming language in their work.
Table of Contents
Google (Alphabet)
The Go programming language is completely open-source, but the language has its origins at Google, so it’s hardly surprising that the company is using it extensively.
The larger company that owns Google is known as Alphabet, due to corporate restructuring that occurred in 2015, but most people understandably still refer to the entire company as Google.
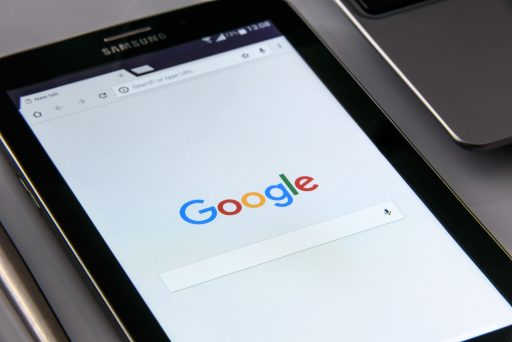
It has dominated the marketplace for many years now.
For example, gRPC is a high-performance, open-source framework for building Remote Procedure Call (RPC) APIs that can be used in scalable microservices. It that was written in Go by developers working at Google, although it is now maintained by the Cloud Native Computing Foundation.
Kubernetes — the very popular container orchestration system that is often used alongside Docker — was also initially developed by Go coders working at Google. The project began as an attempt to solve the challenges that Google was facing in managing its own infrastructure, which involved running thousands of containers across multiple data centres.
Uber
Uber uses Go as a central part of its tech infrastructure. The company runs a microservice written in Go that is used to handle information about certain geographically defined areas known as geofences.
For example, a geofence may be used to define an area where drivers can charge higher prices, because there’s been a sudden surge in demand.
Using geofencing to apply surge pricing (also known, more neutrally, as dynamic pricing) is central to Uber’s business model, but this practice has also generated a certain amount of controversy, especially when higher prices are charged during terrorist attacks or mass shootings, as people rush to escape the affected area for the sake of their own safety.
The ability to let prices quickly reflect demand is, without doubt, a highly efficient and effective system, but some people see it as encouraging unethical profiteering.
Nonetheless, the team at Uber is clearly committed to the Go programming language, so much so that they’ve even produced one of the best style guides available for the language (after Google’s own document, of course).
For example, the Uber style guide recommends starting enums at 1
(rather than the default of zero), so that 0
can be used to signify an invalid value:
package main
import "fmt"
type vehicle uint8
const (
car vehicle = iota + 1
bus
motorbike
hovercraft
)
func main() {
var v vehicle
if v == 0 {
fmt.Println("v is not a valid vehicle")
}
}
We can now safely infer that if a vehicle
variable equals zero, then it has not had a valid value assigned and should be ignored.
Slack
Slack operates a messaging application, originally intended to be used by teams in the workplace, but which has now been adapted also for personal use. It is designed to help people communicate and collaborate with each other more effectively.
There is an official package that allows users to send and receive messages on Slack directly from their Go code.
For example, the following code can be used to send a message to a Slack channel:
package main
import (
"fmt"
"log"
"github.com/slack-go/slack"
)
func main() {
api := slack.New("TOKEN")
channelID, timestamp, err := api.PostMessage(
"CHANNEL_ID",
slack.MsgOptionText("This is an example message.", false),
)
if err != nil {
log.Fatalln(err)
}
fmt.Printf("Message successfully sent to channel %s at %s", channelID, timestamp)
}
As can be seen above, calling slack.New
returns a pointer to a slack.Client
struct, which can then be used to send a message to a given channel.
Repustate
Repustate is a company that provides sentiment analysis, using natural-language processing to analyse text in order to attempt to quantify and classify customer feedback and other written content.
They found that by migrating their entire API stack from Python to Go, the company was able to reduce the average response time of an API call by 1000%, from one hundred milliseconds to ten milliseconds.
The fact that Python is an interpreted language means that it will almost always run slower than a compiled language like Go.
The developers at Repustate were also impressed with the Go compiler’s ability to cross-compile code, allowing executables to be created that will run on many different processor architectures.
Khan Academy
Khan Academy is non-profit educational organization that provides free online educational videos and materials to students and teachers around the world.
Its developers, like those at Repustate, have also switched their primary codebase from Python to Go. This was a monumental task, however, because they were working with half a million lines of code.
They ultimately decided to make the switch because Python 2 was reaching the end of its life in 2020, and a large migration to Python 3 would have been necessary to keep the original codebase active.
This period of upheaval gave them an ideal opportunity to switch to Go, an altogether different language that would clearly be able to outperform both versions of Python.
Some engineers on the development team at Khan Academy have noted that it is precisely because Go has fewer language features than Python that it has become easier and quicker to read other people’s code, which should help other programmers to maintain and update the codebase in the future.
John Resig, who is perhaps most famous for creating the Javascript library jQuery but who now works as Chief Software Architect at Khan Academy, has said the following about the transition:
Go has been instrumental in enabling us to handle millions of requests per day with minimal downtime. Its ease of use, concurrency support and performance make it a natural choice for building scalable backend services.
The only downside noted was that Go code tends to be more verbose than the equivalent code in Python, but it’s precisely that “clunkiness” that makes Go easier to read than other languages that try to be “clever” simply for the sake of terseness.
It’s better to be ugly and understood than beautiful and baffling.
American Express (Amex)
Before choosing to use Golang, the development team at American Express took part in what they grandly called a “Language Showdown”.
This competitive process involved writing the same code in a number of different programming languages and then benchmarking its performance.
Four programming languages were used in this competition: C++, Java, Node.js and Go. The first three languages were already widely used at Amex, but Golang had not yet been adopted by the company.
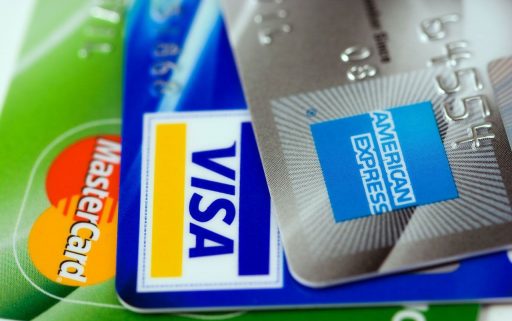
As part of the Language Showdown, Amex’s developers wrote an HTTP service in each of the four programming languages that could convert ISO 8583 messages to JSON.
You may not be aware of the ISO 8583 standard, but it is a binary format widely used by the financial industry to send messages between handheld card-reading devices and distant servers run by the card issuers when, for example, a customer uses a credit card to make a payment in a store.
By focusing on code converting between ISO 8583 and JSON, the developers were ensuring that the winner of the Showdown would have strong support for both older and newer message formats.
When the performance of the HTTP services was analyzed, it was found that Go achieved the second-best performance at 140,000 requests per second — not far from C++, which achieved 167,000 requests per second.
However, the elegance and simplicity of Go’s syntax is what really convinced the Amex team to choose it as the winner of their Language Showdown, since they could be confident that it would be easy to train developers who hadn’t used Go before to write advanced code in the language.
On the other hand, C++ has such a huge standard library and complicated multi-paradigm style that a developer can’t really be considered truly competent in the language until many years have been spent working with it.
British Broadcasting Corporation (BBC)
The BBC is one of the biggest and most respected broadcasters in the UK and around the world.
The BBC’s digital team is responsible for handling between three and four terabytes of live audio and video content, which is played by streaming users with around thirteen million events logged every single day.
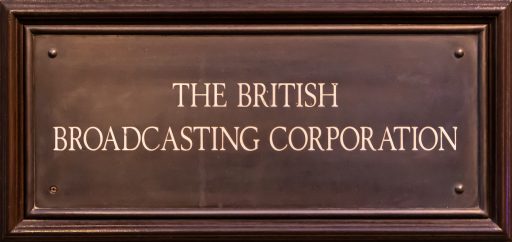
The corporation clearly has to be able to run a huge media operation online, and Go servers play a very large role in this.
Go has also been used at the BBC to build a personalized recommendation engine that suggests TV shows that users may want to watch based on their previous viewing history.
The engine uses goroutines in order to process large amounts of data in real-time, aiming to make personalized recommendations within milliseconds.
Mercari
The Japanese e-commerce company Mercari was only founded in 2013, but it already runs Japan’s largest community-powered marketplace.
If you’re not aware of the platform, perhaps you could think of it as something of a mix between eBay and Craigslist. Billions of dollars worth of transactions take place on it every month.
(The company’s name comes from the ancient Latin word mercari, which means “to trade” or “to complete a business deal”.)
Mercari uses Go code in its backend infrastructure, including its API server. However, perhaps more interesting is the way that it also makes use of the programming language in the AWS Lambda “serverless” computing platform, which allows developers to write and deploy functions that run in response to named events, without needing to set up and manage their own server infrastructure.
The AWS Lambda platform uses Docker containers to provide a lightweight and flexible runtime environment for the event-driven functions. Even though the platform itself is written in Go, developers have the option to write functions in Python, Ruby, Java, C# or Node.js, as well as Go, and deploy them using a simple command-line tool.
The example below shows a simple Go function that could be deployed to AWS Lambda:
package main
import (
"encoding/json"
"log"
"github.com/aws/aws-lambda-go/lambda"
)
type Event struct {
Name string `json:"name"`
}
func HandleRequest(e Event) (string, error) {
return fmt.Sprintf("Hello, %s!", e.Name), nil
}
func main() {
lambda.Start(HandleRequest)
}
The HandleRequest
function will run whenever a JSON-formatted document that has a "name"
field is passed to the service, returning a friendly greeting in response.