Can You Identify the National Flags of the World?
Language
- unknown
by James (Golang Project Structure Admin)
I wanted to create a fairly simple game, which would be fun to play but quick to build, so that I could upload it to Itch, the popular website that allows users to host their own indie games. My intention was to try and find out how easy — or hard — the process would be and see whether I was happy with the finished result and the overall service provided by Itch.
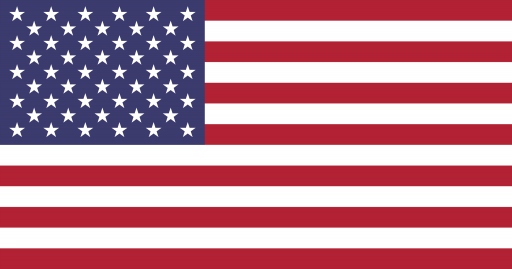
So I created a basic trivia-based memory game, where players have to match a certain number of national flags with the correct country for each. The end result is called Know Your Flags and it’s available to play now.
Table of Contents
How I Created the Game
Even though I love Golang, I have to admit that this game isn’t written in the programming language that I use most often. I do, however, plan to write future games in Go, compiled down to WebAssembly, so that they run natively in the browser.
This game is written in Typescript, using a homemade 2D game engine that I’d put together in the past: that little engine is probably overkill for such a simplistic game, since I don’t use any of its drawing capability. The game uses nothing other than a few HTML elements to display everything on the screen.
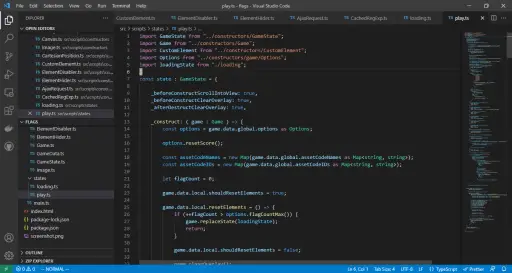
Even so, the engine did help me in other ways: for example, it allowed me to easily make use of two different game states, one for the entry screen and another for the main game logic. This is important, because it allowed me to focus on keeping my code organized, ensuring that separate functionality stays in separate data structures and files.
The main state is pushed onto a stack and then popped off when the gameplay has finished, returning the player to the original view — so that he or she can choose to quit or restart the game, after having the chance to modify the difficulty settings, if the whole thing seemed too hard the first time around.
Each of the state objects implements the following interface (although the real interface has even more methods, in order to handle various edge cases):
GameState.ts (partial extract)
export default interface GameState {
_construct? : ( game : Game ) => void;
_destruct? : ( game : Game ) => void;
_update? : ( game : Game ) => void;
_draw? : ( game : Game ) => void;
_onResize? : ( game : Game ) => void | boolean;
_onMouseMove? : ( game : Game ) => void | boolean;
_onMouseDown? : ( game : Game ) => void | boolean;
_onMouseUp? : ( game : Game ) => void | boolean;
_onClick? : ( game : Game ) => void | boolean;
_onDoubleClick? : ( game : Game ) => void | boolean;
_onKeyDown? : ( game : Game ) => void | boolean;
_onKeyUp? : ( game : Game ) => void | boolean;
}
Within the Game
class, which is used to manage everything on the screen, the following methods can be used to add and remove (push and pop) states:
Game.ts (partial extract)
export default class Game {
private _stateStack : Array = [];
public pushState( state : GameState ) : void {
if (this._stateStack.length > 0) {
this._runDestruct();
}
this._stateStack.push(state);
this._runConstruct();
}
public popState() : void {
if (this._stateStack.length === 0) {
return;
}
this._runDestruct();
this._stateStack.pop();
if (this._stateStack.length > 0) {
this._runConstruct();
}
}
public replaceState( state : GameState ) : void {
const lastIndex = this._stateStack.length - 1;
if (lastIndex >= 0) {
this._runDestruct();
this._stateStack[lastIndex] = state;
} else {
this._stateStack.push(state);
}
this._runConstruct();
}
public peekState() : GameState | null {
const stateStack = this._stateStack;
const stateIndex = stateStack.length - 1;
return ((stateIndex < 0) ? null : stateStack[stateIndex]);
}
}
Beginning the Game: the Initial Screen
The only thing you have to do before you start to play is choose how hard you want the gameplay to be.
There are varying levels of difficulty, from “Very Easy” to “Very Hard” and even “Genius”, but, by default, the game loads in the “Medium” setting, which should give a good degree of difficulty for most players.
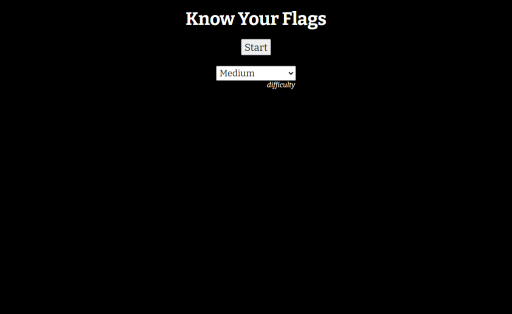
If you want to start on the “Very Easy” level, which will give you fewer multiple-choice options, just select it from the drop-down list. Once you complete the game with a reasonable score on a certain difficulty-level, you can always make it harder, which means that there should always be an increasingly tricky challenge available, until you’ve mastered your reaction times and memorized all of the flags that can possibly appear.
How to Play the Game
When you press the “Start” button, the game will begin immediately.
You will then be shown an image of a national flag underneath the header that contains the text “Know Your Flags”, the game’s title. It’s your job, of course, to identify which country the currently visible flag belongs to.
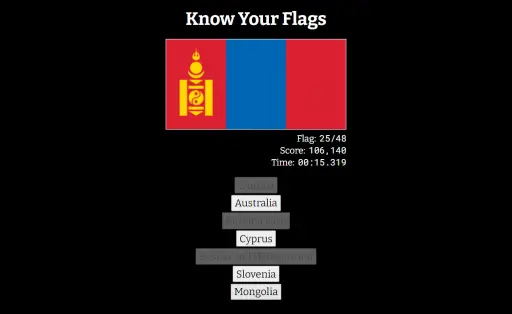
In the screenshot above, the text “Flag: 25/48”, shows how many flags I’ve identified and how many in total I need to identify before the game is completed: since I was on the 25th flag out of a total of 48, I was just over halfway through the game when this image was taken.
Next the score is displayed, which is calculated differently depending on the difficulty you’ve selected. In general, if you identify the correct answer, you’ll be awarded points, but if you choose an incorrect option, you could be deducted points, which can even cause your score to become a negative number, especially when you’re playing with one of the harder difficulty options.
The final text to be shown is a timer, showing minutes, seconds and milliseconds. This starts when a national flag is first displayed on the screen. If the image is slow in loading, the timer will not start until it has fully loaded, so don’t worry about a slow internet connection affecting your game. However, this timer may influence your final score, because you may be rewarded with more points if you identify the correct answer quickly than if you take lots of time to think about it.
How Many Flags Does the Game Contain?
The game currently contains over 250 different flags. This is more than the number of different countries in the world. According to Wikipedia, there are 206 sovereign states (in other words, independent nations that are each ruled by their own government).
However, Know Your Flags also includes the flags of supranational entities like the United Nations and the European Union. In addition, there are the flags of territories that aren’t technically independent states, but which still have their own unique history and identity, like New Caledonia, Greenland and the Isle of Man.
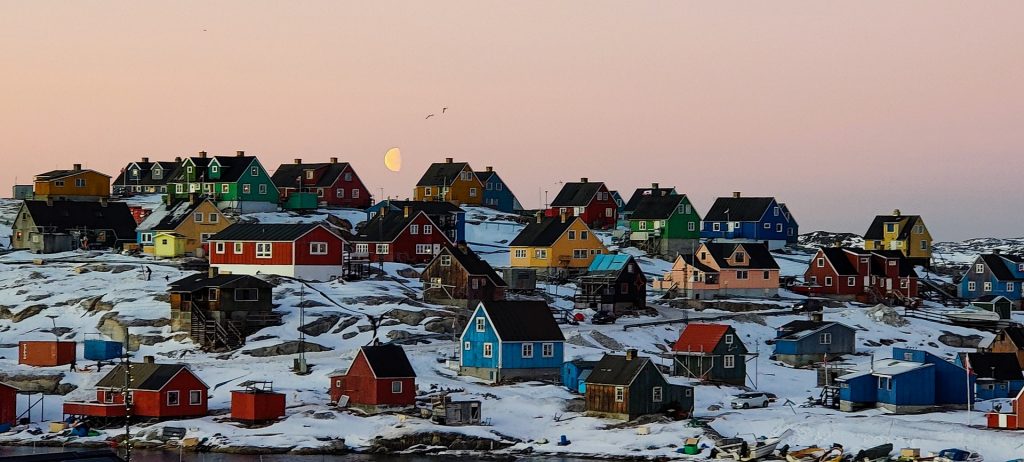
You won’t be shown all of the available flags when you play the game, however. If you begin in “Very Easy” mode, you will only have to identify eight different flags, and you’ll be given a choice of four different multiple-choice options for each flag, where one of them is the correct answer. If you decide to take on the immense challenge of “Genius” mode, on the other hand, you will be expected to identify 240 different flags, and you’ll have nine different options per flag to choose from.
What I Think About Itch
I was really impressed by the service provided by Itch. Uploading an HTML game like this was really easy: I was able to provide a zip file that contained all the code, and this would then be uncompressed and hosted at its own URL on the company’s servers.
The assets — in this case, the various images of national flags — could simply be stored within their own subdirectory and accessed via a relative path from the Typescript code that had been transpiled down to Javascript, allowing the game to run identically both on my own computer and on Itch.
When the game is viewed online, the content is actually in an iframe
. This is nice because it keeps the game’s visuals separate from Itch’s own branding and user interface that surrounds it. However, the iframe
must be set to a static size, which makes it more difficult to create a responsive experience for smartphones and tablets. This is something I’d like to see improved, but it isn’t a major problem, since the user can always press the button in the bottom-right corner to enter full-screen mode, if they wish.
As you can see in the screenshot above, it is also possible for users to give monetary donations to the authors of games, if they like what they’ve just played or appreciate the effort that went into creating it. This is an awesome feature, since it helps developers to understand how players feel about the game — and it also goes some way towards supporting the costs that inevitably go into creating well-crafted code. Just a few dollars can give a huge amount of encouragement to someone and make them feel like you really appreciated their work.
If you do want to earn money from your games, then you’d probably do better to release them on Steam, the App Store for Apple iOS or Google Play for Android, which are marketplaces with much larger audiences that have a long history of being willing to engage with a pay-to-play model of software publishing. However, if you just want to earn a bit of extra cash, while releasing a fun little game that you’ve been working on in your spare time, then Itch is absolutely perfect: I can’t recommend it highly enough!
Enough Talk, I Just Want to Play the Game
Click this link to play Know Your Flags on Itch right now. Let me know if manage to achieve a spectacularly high score. I’ll be extremely impressed if anyone manages to complete the game in “Genius” mode with a positive score, because I’ve tried many times and never succeeded.
Feel free to get in touch if you have any feedback or constructive criticism about the game, too. It was just a small project that I spent half a day coding, but I’d love to hear what you think.