Removing a Substring From a String in Go
Language
- unknown
by James Smith (Golang Project Structure Admin)
In this blog post, we will explore several approaches that can be used to remove a substring from a larger string in Go, covering both basic cases and more complex scenarios.
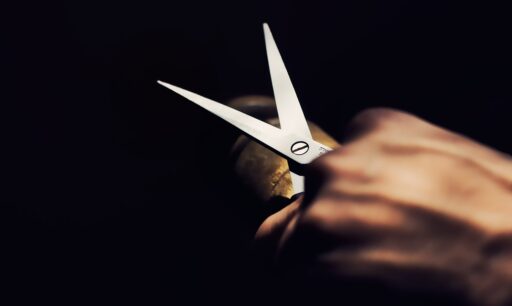
By the end of this guide, you should have a solid understanding of various ways to strip substrings from strings in Go and how to apply these techniques in real-world applications.
Table of Contents
Prerequisite Reading
This is the second part of a two-part post about dealing with substrings in Go.
The first post discusses how to locate a substring within a string and it is available to read here.
Considering Some Common Use Cases for Removing Substrings
Before diving into the implementation details, it may be helpful to explore some real-world situations where removing a substring from a string can come in useful.
One common use case is sanitizing input given by users of a program. In web applications, for example, you may need to filter out specific characters, words or text to clean the input, such as removing offensive language or stripping away certain HTML tags. This filtering may be important for maintaining security and a good user experience.
Another scenario involves data cleaning. When working with large datasets, it’s typical to encounter extraneous and unnecessary information like unwanted punctuation, excess whitespace or special formatting characters. These substrings can clutter the data, so removing them is a crucial step in preparing the dataset for analysis.
String formatting in general is an area where removing substrings can come in handy. Strings from various sources often need to be standardized, especially when dealing with URLs, file paths or other forms of structured text. By removing specific substrings, you can ensure consistency and avoid potential errors when working with these strings.
Finally, log parsing frequently involves removing unimportant information to extract the relevant content. When analyzing log files, you may need to strip away timestamps, unique IDs or other unnecessary details to focus only on the meaningful data for debugging or monitoring purposes.
Using the Go Standard Library’s Replace Function
The simplest and most common way to remove a substring from a string in Go is by using the strings.Replace
function, which is part of the language’s standard library.
This function allows you to replace all occurrences (or a limited number of occurrences) of a substring with another string.
Of course, if we just want to remove a substring, then we simply need to replace it with an empty string (""
).
Looking at How to Call the Replace Function
The call signature of the strings.Replace
function in Go is as follows:
func Replace(s, old, new string, n int) string
Here, s
refers to the original string that we want to use to make replacements.
The second parameter, old
, represents the existing substring that you want to replace within the s
string.
The third parameter, new
, specifies the string that we want to replace the old substring with.
As we mentioned, if you intend to remove the substring entirely, then this parameter should be set to an empty string.
The final argument, n
, determines the number of occurrences to replace.
If we want to replace all occurrences of the substring, we can set n
to -1
(or any other negative value), which will set the function to remove as many instances of substring as it finds within the larger string.
Example of Using the Replace Function to Remove All Occurrences of a Substring
Let’s look at an example using the strings.Replace
function now:
package main
import (
"fmt"
"strings"
)
func main() {
s := "Hello, Go! Welcome to Go!"
result := strings.Replace(s, "Go", "", -1)
fmt.Println(result)
}
In the example above, we replace all instances of "Go"
with an empty string, effectively removing the substring from the larger string.
As a result, the code should output the following text:
Hello, ! Welcome to !
Removing a Substring Only Once
If we had wanted to remove only the first occurrence of the substring, we could have passed 1
as the last argument to the strings.Replace
function, as shown below:
result := strings.Replace(s, "Go", "", 1)
fmt.Println(result)
This would have removed only the first occurrence of the substring "Go"
, thereby producing the following output:
Hello, ! Welcome to Go!
Using the Builder Struct to Create a Shorter String
While the strings.Replace
function is convenient, it may not always be the most efficient option, particularly when dealing with large strings or more complex forms of string manipulation.
In such cases, we can use the strings.Builder
type to efficiently build a new string by appending segments of the original string — while omitting the parts that we want to remove.
Why Would We Choose to Use a Builder?
One key advantage of using strings.Builder
is its efficiency.
Remember that, in Go, strings are always immutable, meaning that every time you modify a string, a new one is created in memory.
This process can be inefficient, especially when working with very large strings or performing a large number of string modifications.
By contrast, strings.Builder
provides a way to construct strings more efficiently, as it allows us to append content without constantly allocating new memory for each modification.
Using strings.Builder
also gives us greater control over the string-building process, which is particularly useful for more complex or iterative string manipulations.
You can append different elements, format content or concatenate substrings in an optimized way by making use of a strings.Builder
.
Example of Using a Builder to Remove a Substring
Now here’s an example where we manually remove a substring using the strings.Builder
type:
package main
import (
"fmt"
"strings"
)
func main() {
s := "Hello, Go! Welcome to Go!"
substring := "Go"
var builder strings.Builder
// iterate over the original string and append to the builder
for i := 0; i < len(s); {
if strings.HasPrefix(s[i:], substring) {
i += len(substring) // skip over the substring
} else {
builder.WriteByte(s[i]) // append the current character
i++
}
}
result := builder.String()
fmt.Println(result)
}
In the code above, we enter a for
loop that iterates over the original string, character by character.
At each iteration, we check if the current position of the string begins with the substring "Go"
by using the strings.HasPrefix
function. This function determines whether the substring is found starting from the current index, i
, of the original string.
If the substring is found, we skip over it by increasing the index i
by the length of the substring.
This effectively ignores the substring and prevents it from being added to the final result.
However, if the substring is not found at the current index, we append the current character to the strings.Builder
using the builder.WriteByte
method. This method appends a single byte (or ASCII character) to our builder.
After the loop has completed its work, we convert the contents of the strings.Builder
to a string by calling the builder.String
method.
This gives us the final result where all occurrences of "Go"
have been removed.
The output is then printed, displaying the modified string "Hello, ! Welcome to !"
, which we also saw earlier when we used the strings.Replace
function.
Removing Substrings With Regular Expressions
Regular expressions provide a more powerful method for string manipulation, allowing us to match patterns and remove substrings dynamically.
In Go, the "regexp"
package provides tools for performing regex-based string operations.
The "regexp"
package is particularly useful when handling more complex patterns, where you may need to match and remove text based on rules that go beyond simple substring matches.
Looking at How to Call the ReplaceAllString Function
The regexp.ReplaceAllString
method, which is called on a previously compiled regular expression, can be used to remove substrings that match a specific pattern.
Below is its call signature:
func (re *Regexp) ReplaceAllString(src, repl string) string
Here the src
parameter represents the source string that we want to modify, while the repl
parameter specifies the replacement string.
As before, if we want to remove the matching substrings entirely, then we can set repl
to an empty string. This effectively replaces any substring that matches the regular expression with nothing, thereby removing it from the source string.
Example of Removing All Digits From a String
Let's say that we want to remove all of the digits from a string — perhaps we want to remove personally identifying information like credit-card numbers or telephone numbers from some text.
In this case, we can use a regex pattern, such as "\d"
, which is used to match any single digit.
Now here's a code example:
package main
import (
"fmt"
"regexp"
)
func main() {
s := "User123 has 954 points!"
re := regexp.MustCompile(`\d`)
result := re.ReplaceAllString(s, "")
fmt.Println(result)
}
In the example shown above, we use a very simple regular expression to find and remove each of the digits from the original string, which results in the following numberless output:
User has points!
Removing Multiple Patterns
Of course, we can also use regular expressions to match and remove multiple patterns at once.
For instance, we could have chosen to remove both digits and some common punctuation marks from our previous string like so:
re := regexp.MustCompile(`[0-9,.!?]`)
result := re.ReplaceAllString(s, "")
Removing Substrings Based on Dynamic Conditions
However, in some situations, you may need to remove substrings based on more dynamic and programmatic conditions.
It's possible that you could want to remove all occurrences of substrings that match a certain criterion — for example, all substrings that start with a specific prefix.
Here’s a code example where we remove all words starting with the prefix "temp"
from within a string:
package main
import (
"fmt"
"strings"
)
func main() {
fileNames := "tempFile1 tempFile2 permanentFile"
words := strings.Fields(fileNames)
var builder strings.Builder
for _, word := range words {
if !strings.HasPrefix(word, "temp") {
builder.WriteString(word + " ")
}
}
result := strings.TrimSpace(builder.String())
fmt.Println(result)
}
In this code, the string "tempFile1 tempFile2 permanentFile"
is first split into individual words using the strings.Fields
function.
This function breaks the string up by looking for whitespace, returning a slice of individual words.
We then iterate over the slice, checking each word to see if it begins with the prefix "temp"
using the strings.HasPrefix
function.
If a word does not start with the prefix "temp"
, it is appended to the strings.Builder
.
After all of the words have been processed, the result is converted to a string, and we use the strings.TrimSpace
function to remove any trailing spaces that might have been added during the building process.
Removing Substrings at Specific Positions
In the previous examples, we've been assuming that we haven't already found the substring when we need to remove it.
However, if you know the specific position (i.e index) within the string where the substring is located, you can use Go's slicing techniques to create a new string that excludes the desired substring.
Let’s say you want to remove a substring that's found between index 7 and index 9 from the string "Hello, Go!"
, as in the code below:
package main
import "fmt"
func main() {
s := "Hello, Go!"
start := 7
end := 9
result := s[:start] + s[end:]
fmt.Println(result)
}
In this example, we take the part of the string before index 7 and the part after index 9, then concatenate the sliced strings to form the new string "Hello, !"
with the substring "Go"
removed.
Optimizing String Manipulation for Performance
String manipulation can sometimes be a resource-intensive process, especially when done in bulk.
Below are some key optimization techniques to consider when removing substrings in Go.
Use a Builder
As we mentioned earlier, strings.Builder
is a highly efficient way to build strings dynamically.
It avoids the performance overhead that comes from repeatedly creating new strings at each step of modification.
This is particularly beneficial when you need to construct or modify strings in multiple steps.
Avoid Repeated String Modifications
Since Go strings are immutable by their very nature, every time you modify a string, a new string gets created in memory.
To mitigate this, try to batch multiple modifications into a single loop or operation, minimizing the number of new string allocations and thus reducing memory overhead.
Use Byte Slices
For more complex string manipulations, converting the string into a byte slice ([]byte
) can be more efficient.
Byte slices are mutable, unlike strings, which means that you are able to modify them without creating new copies of the data.
After performing the necessary operations to modify the data, you can then convert the byte slice back into a string, as shown below:
codes := []byte("Hello, Go!")
// perform operations on the byte slice
result := string(s)
When a string is initially converted to a byte slice, new memory will be allocated. However, when a byte slice is converted to a string, no new memory is allocated, since Go will reuse the underlying data of the byte slice when constructing the string.
Use Regular Expressions Judiciously
Regular expressions clearly offer a powerful way to search through and manipulate strings, but they can also introduce an additional degree of performance overhead, especially when handling complex patterns.
While they are useful for pattern matching, try to use regular expressions only when truly necessary and avoid overly intricate patterns that could cause your application to slow down.
In many cases, simpler string functions like strings.Contains
or strings.HasPrefix
may suffice, while also offering better performance.